Insights
Beginner's introduction to React.js
2 min read.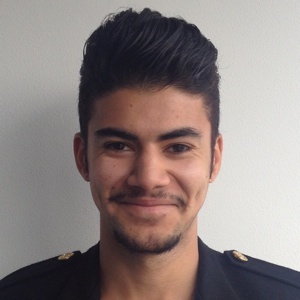
Pablo Vallejo
Software Engineer
With the growing number of JavaScript libraries and architectural approaches it becomes really difficult to figure out which one would suit our needs, and even whether we need to use one of these or not.
Back in 2013 Facebook released a front-end framework called React that they were using internally for managing user interface updates within their apps. React is mainly thought of as the view part in the MVC model. Its main function is to bind HTML elements to data and once this data changes, React takes care of updating the interface so that you don't have to bind this data manually.
In this article, we're going to explain the simple to-do app from the react tutorial, which is composed of two things, the first, a list of the current to-do items and the second, a form for adding a new to-do. New items are added to the list once we submit the form.
Project setup
For the sake of simplicity, the project is going to be a HTML file with a JSX script.
Main file index.html
<!DOCTYPE html>
<html>
<head>
<script src="http://fb.me/react-0.12.2.js"></script>
<script src="http://fb.me/JSXTransformer-0.12.2.js"></script>
<style>.container{max-width: 960px; margin: 0 auto; }</style>
</head>
<body>
<div class="container">
<div id="todoExample"></div>
</div>
<script src="todo-app.jsx" type="text/jsx"></script>
</body>
</html>
Script file todo-app.jsx
var TodoList = React.createClass({
render: function() {
var createItem = function(itemText) {
return <li>{itemText}</li>;
};
return <ul>{this.props.items.map(createItem)}</ul>;
}
});
var TodoApp = React.createClass({
getInitialState: function() {
return {items: [], text: ''};
},
onChange: function(e) {
this.setState({text: e.target.value});
},
handleSubmit: function(e) {
e.preventDefault();
var nextItems = this.state.items.concat([this.state.text]);
var nextText = '';
this.setState({items: nextItems, text: nextText});
},
render: function() {
return (
<div>
<h3>TODO</h3>
<TodoList items={this.state.items} />
<form onSubmit={this.handleSubmit}>
<input onChange={this.onChange} value={this.state.text} />
<button>{'Add #' + (this.state.items.length + 1)}</button>
</form>
</div>
);
}
});
React.render(<TodoApp />, document.getElementById('todoExample'));
What is JSX?
JSX is a XML-like syntax extension to JavaScript that allows us to define tree structures with attributes. These JSX scripts need to be compiled to JavaScript in order to be used in the browser.
Sample JXS code
var TodoList = React.createClass({
render: function() {
var createItem = function(itemText) {
return <li>{itemText}</li>;
};
return <ul>{this.props.items.map(createItem)}</ul>;
}
});
Compiled EXS code
var TodoList = React.createClass({displayName: "TodoList",
render: function() {
var createItem = function(itemText) {
return React.createElement("li", null, itemText);
};
return React.createElement("ul", null, this.props.items.map(createItem));
}
});
render
method
The render method takes care of rendering a React element into the passed container.
>React.render(<TodoApp />, document.getElementById('todoExample') /* specified DOM container */);
Attributes items
, onSubmit
, onChange
These methods are defined as attributes of the DOM node and are called after a specific event.
items: list of to-dos that get rendered.
onSubmit: calls handleSubmit
after the form is submitted.
onChange: calls onChange
once the input value changes.
Wrapping up
Overall, the app workflow goes this way
- We render the
TodoApp
component which renders theTodoList
component as well. TheTodoList
component paints an unordered list with the current items. - Then, the form is rendered and the events
onSubmit
andonChange
are bound. - Now, every time we submit the form, the state is changed which causes the component to re-render showing the new added to-do.
Conclusion and further reading
React.js is a great framework when we want DOM elements to get updated on data changes. React does this job in an optimal way because of its virtual DOM.
Another point is that you can integrate React with an existing framework within your application. Lets say you use Backbone, then, React can take care of updating the DOM every time your model attributes change.
React components are very similar to Web components so, it might be good to get to know then.
Written by Pablo Vallejo
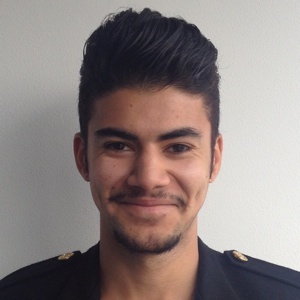
Pablo develops and optimizes software solutions, focusing on functionality and user experience. His expertise in coding and problem-solving ensures the creation of efficient and reliable applications.