How to get a list of most popular pages from Google Analytics in Python?
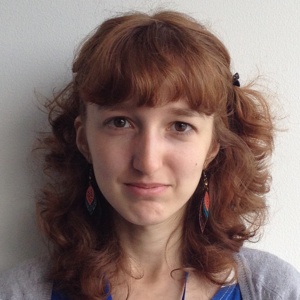
Vera Mazhuga
Software DeveloperLet's look at how to get a list of most popular pages from Google Analytics using Python (Django).
Let's suppose that we have a website and we want to show in our home page a list of 10 most visited pages of our website for the last month.
Create a project in Google Analytics and obtain a tracking code
Firstly, we need to create a project in
Google Analytics and obtain a code to place on all pages of our website.
Go to
Google Analytics website and enter the Admin section:
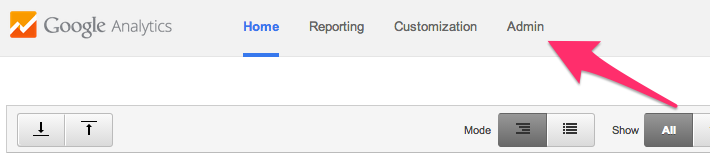
Then you'll need to create a new property for your account:
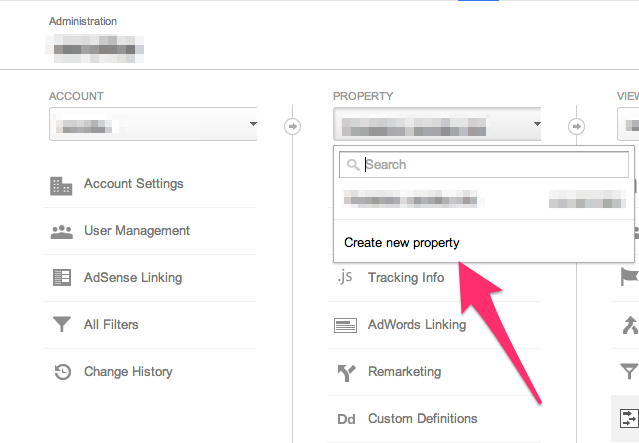
Enter a data related to your website: name, url, category and a timezone:
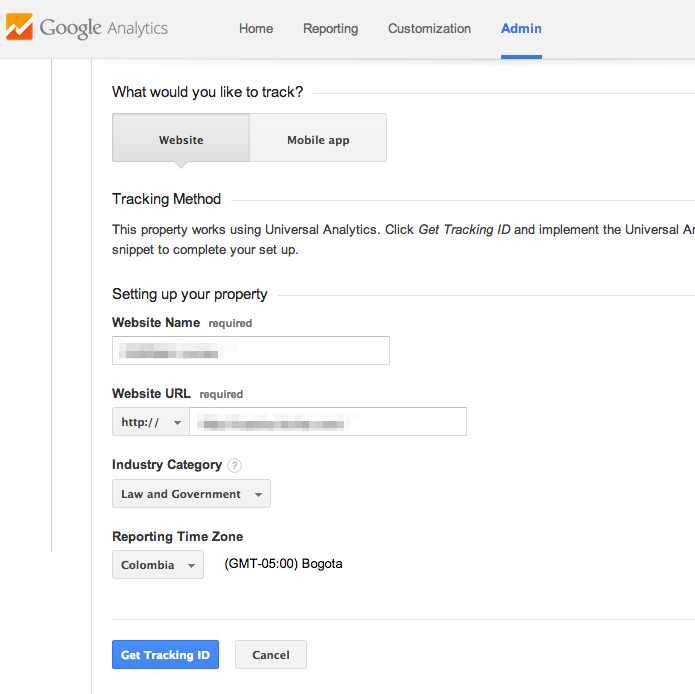
The you need to copy your tracking code and include it to all pages of your website:
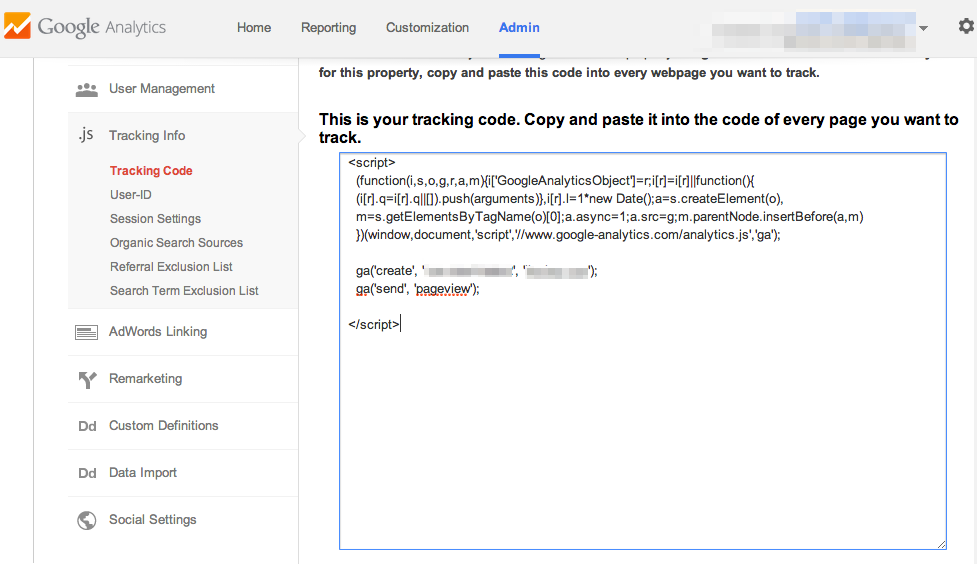
Create a project in Google Console and get a secret key
Then we'll need to enter a
Google Console and create a new project:
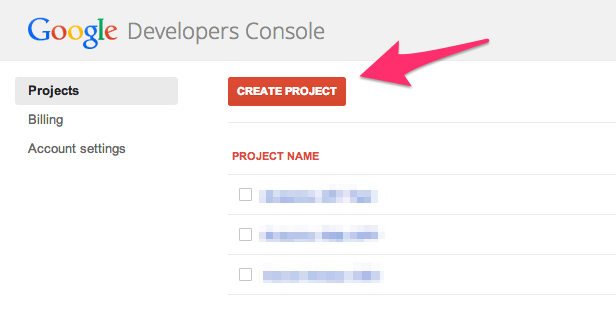
Then we'll need to activate Google Analytics API for our project:
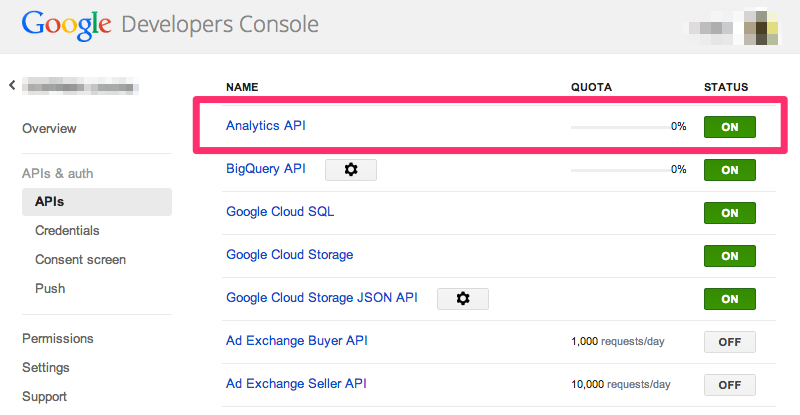
Next, we'll create a new client ID:
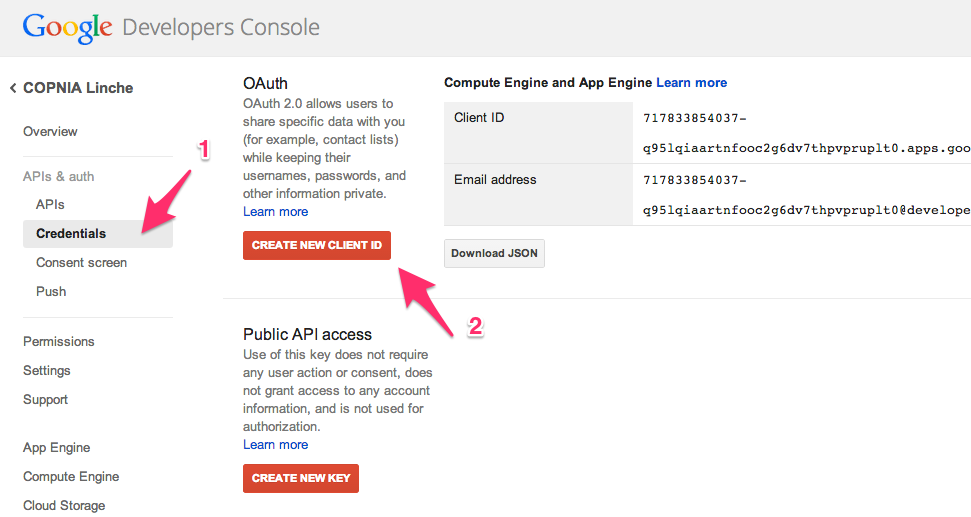
Since we are planning to connect in "offline" mode (via Python code), we'll select a
Service account:
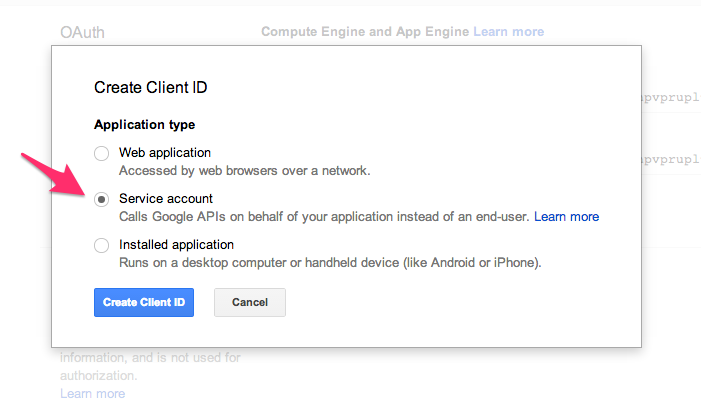
Right after clicking on "Create Client ID" button, we'll get file "
.p12" with a secret code:
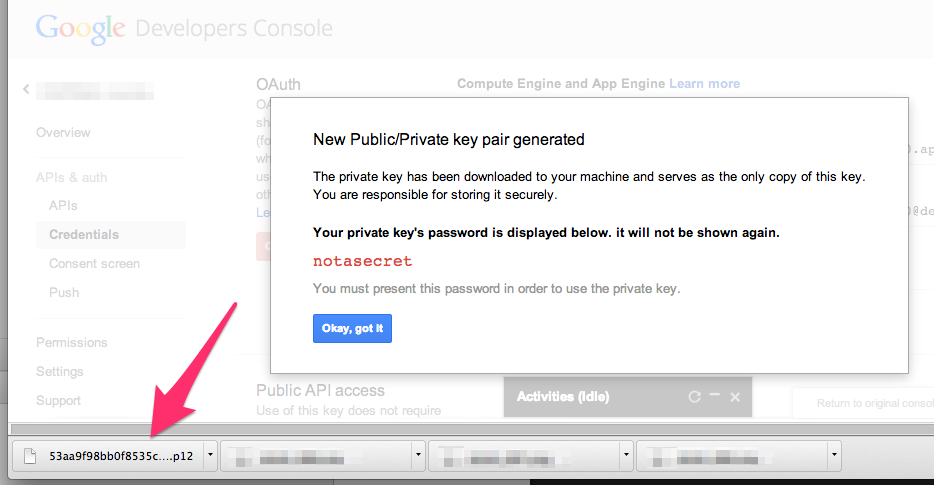
And email that we'll use to connect to API via our Python code:
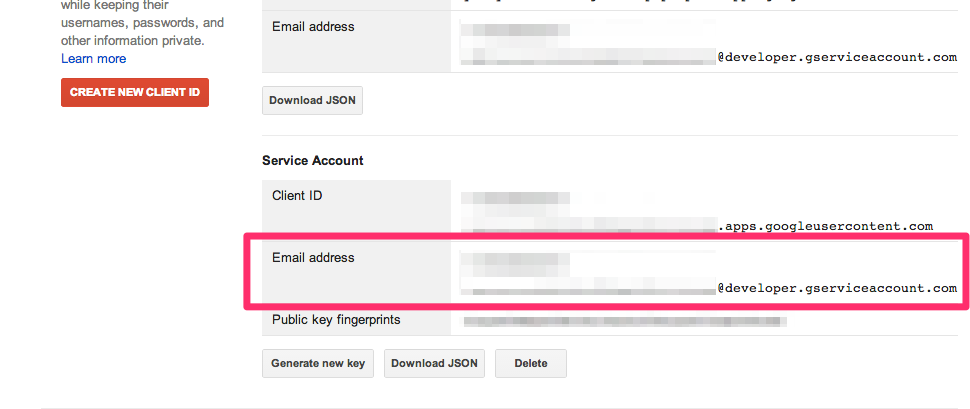
Allow access to Google Analytics
Since we have a "
...@developer.gserviceaccount.com" email, we can add it to the list of users that can have access to Analytics of our website:
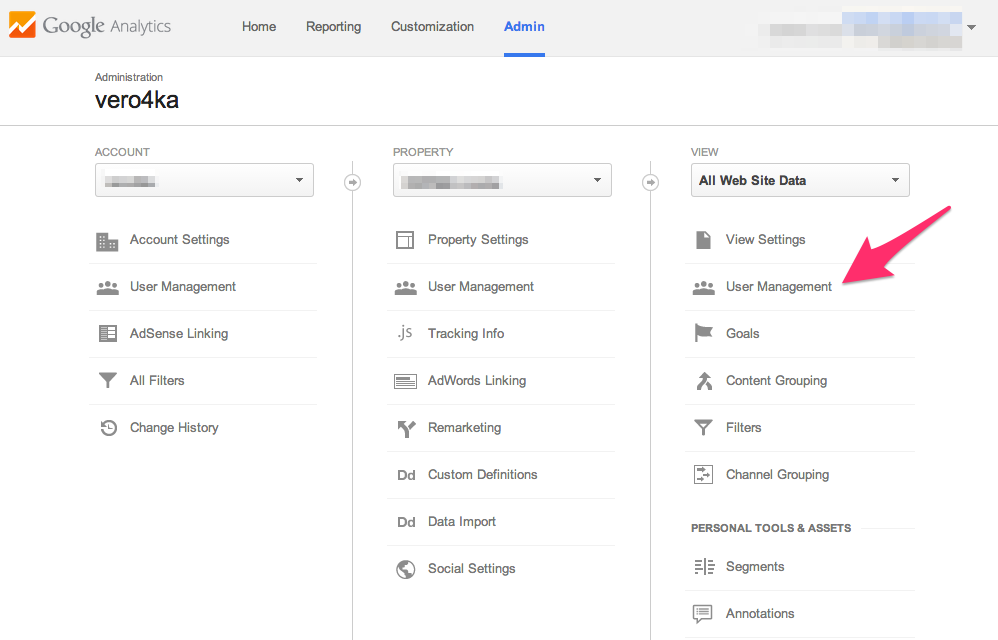
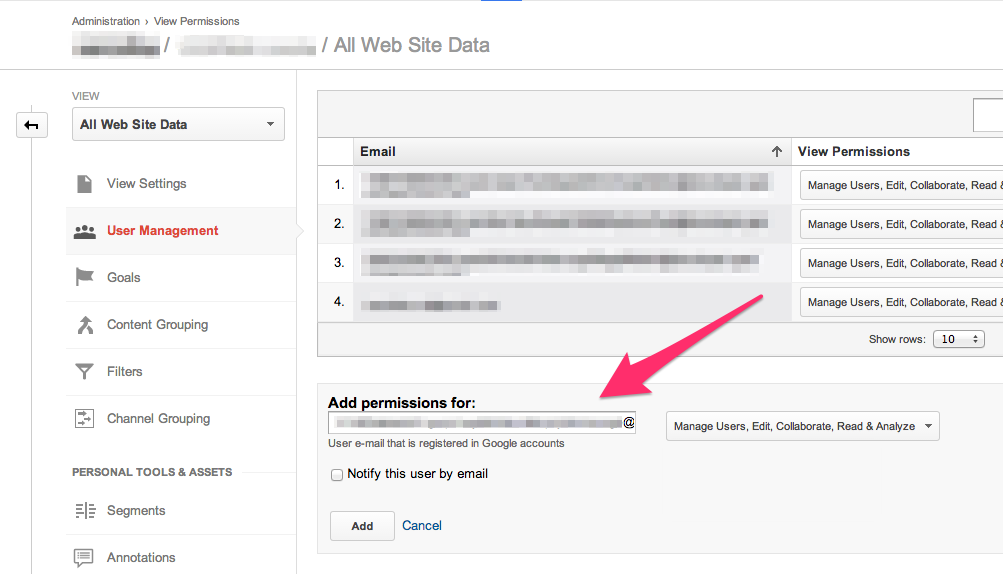
The last thing we need to do on this step is to copy the ID of our project from Google Analytics:
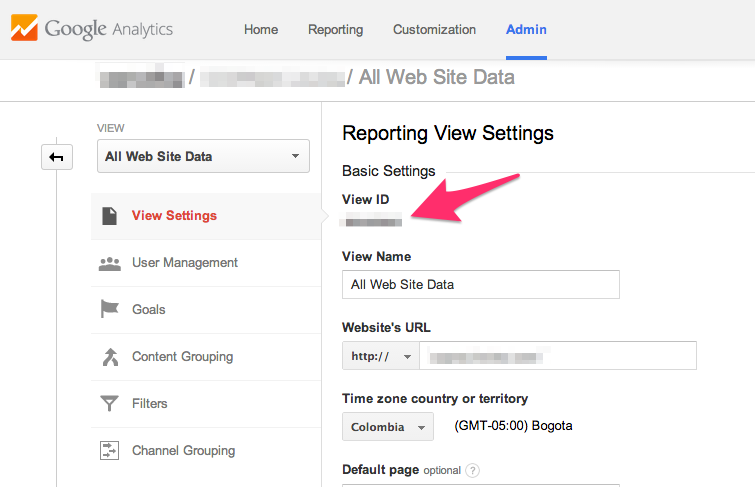
Getting data from Python code
In order to be able to access
Google Analytics API from our Python code firstly, we need to install the following packages: google-api-python-client
, oauth2client
and python-gflags
.
import httplib2
import os
import datetime
from django.conf import settings
from apiclient.discovery import build
from oauth2client.client import SignedJwtAssertionCredentials
# Email of the Service Account.
SERVICE_ACCOUNT_EMAIL ='12345@developer.gserviceaccount.com'
# Path to the Service Account's Private Key file.
SERVICE_ACCOUNT_PKCS12_FILE_PATH = os.path.join(
settings.BASE_DIR,
'53aa9f98bb0f8535c34e5cf59cee0f32de500c82-privatekey.p12',
)
def get_analytics():
f = file(SERVICE_ACCOUNT_PKCS12_FILE_PATH, 'rb')
key = f.read()
f.close()
credentials = SignedJwtAssertionCredentials(
SERVICE_ACCOUNT_EMAIL,
key,
scope='https://www.googleapis.com/auth/analytics.readonly',
)
http = httplib2.Http()
http = credentials.authorize(http)
service = build('analytics', 'v3', http=http)
end_date = datetime.date.today()
# 30 days ago
start_date = end_date - datetime.timedelta(days=30)
data_query = service.data().ga().get(**{
'ids': 'ga:123456', # the code of our project in Google Analytics
'dimensions': 'ga:pageTitle,ga:pagePath',
'metrics': 'ga:pageviews,ga:uniquePageviews',
'start_date': start_date.strftime('%Y-%m-%d'),
'end_date': end_date.strftime('%Y-%m-%d'),
'sort': '-ga:pageviews',
})
analytics_data = data_query.execute()
return analytics_data
You can experiment with different query options using
Google Analytics Query Explorer.
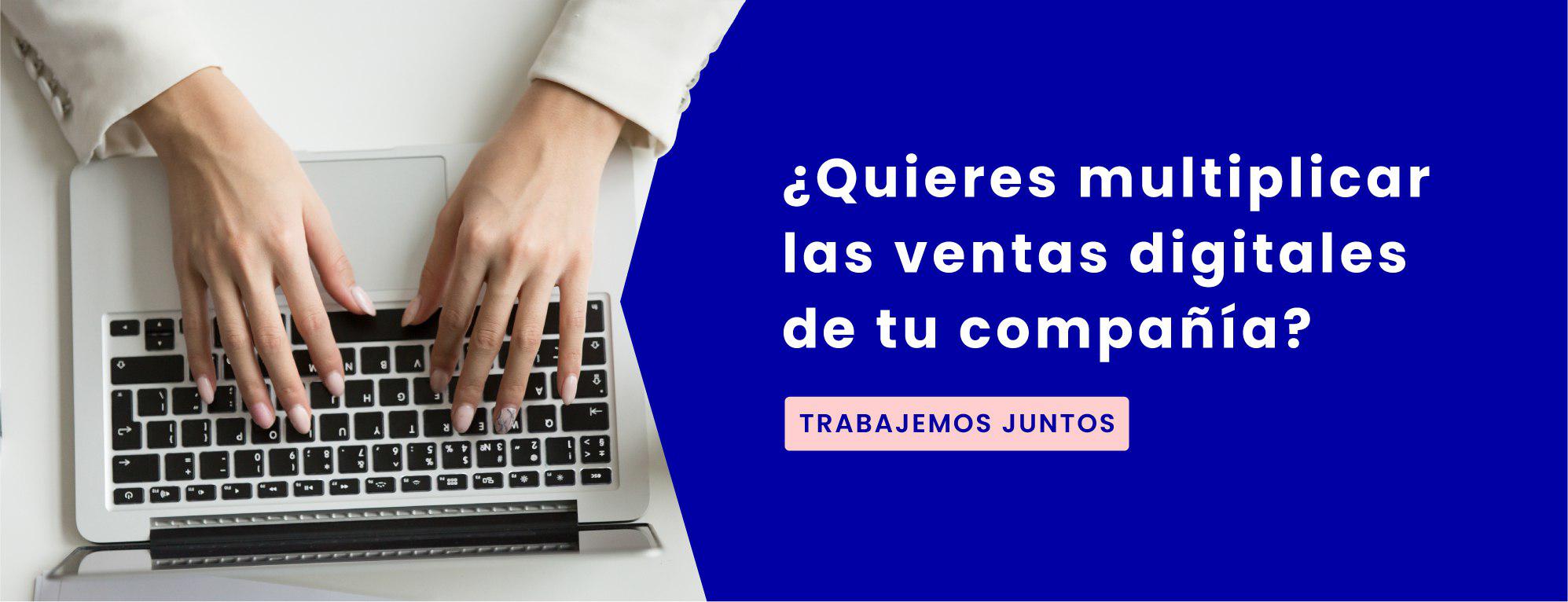
Image was taken from NASA.
Written by Vera Mazhuga
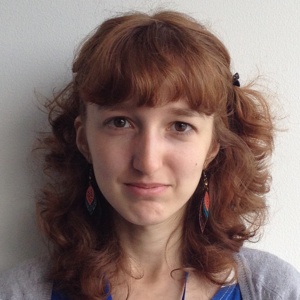
Vera specializes in writing and maintaining code for various applications. Her focus on problem-solving and efficient programming ensures reliable and effective software solutions.