Insights
Introduction To JavaScript Unit Testing Using QUnit
3 min read.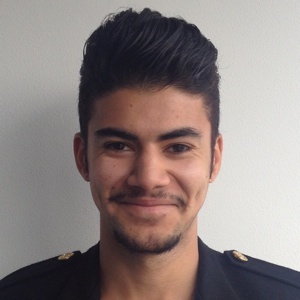
Pablo Vallejo
Software Engineer
During the last years, web applications have become more interactive, and that job no longer rely on Flash, instead, we tend to use JavaScript which has proven to be powerful, flexible and have the capability to let us create pretty much anything we can imagine not only in browser but in the desktop.
Using JavaScript, we can create fancy animations, make AJAX requests, data models and more, but how do we tests them?
In this article, we want to take a look at how to test JavaScript applications using
QUnit which is a simple yet fully featured testing framework originally created by John Resig
Get example code on GitHub:
View on GitHub
What is Unit testing?
Unit testing, is the process of writing code that checks other code is running as it should and returning what we expect it to. In JavaScript, there are several flavors of testing methodologies. Among the most used ones we have TDD (
Test Driven Development) and BDD (Behavior Driven Development).
In
TDD the idea is that we write unit tests before we write the application, in that way we define what we want the code to return and what we want it to do and then we write our actual code based on the tests.
Using QUnit
In this example we will write tests for a calculator object that has four methods:
sum
, subtract
, divide
and multiply
, then we will actually create the calculator object and make sure that it passes all tests we wrote.
Writing calculator tests.
tests/tests.js
/***Calculator tests***/$(document).ready( function() { // Get calculator object var calc = window.Calculator; // Test `sum` method test('Test calculator.sum', 2, function(){ equal( calc.sum( 1, 2 ), 3 ); equal( calc.sum( 5, 5 ), 10 );}); // Test `subtract` method test('Test calculator.subtract', 3, function(){ equal( calc.subtract(5, 5),0); equal(calc.subtract(10, 1),9); ok(calc.subtract(10000,0)); }); // Test `divide` method test('Test calculator.divide', 2, function(){equal(calc.divide(10, 2), 5); equal(calc.divide(5, 5),1);}); // Test `multiply` method test('Test calculator.multiply', 3, function(){ equal( calc.multiply(2, 2),4); equal(calc.multiply(4, 4),16); notEqual(calc.multiply(9, 9),60);});});
ok()
: This method checks whether everything works without throwing an error.
equal()
: Assert that the two passed parameters are the same.
notEqual()
: Tests that the two passed parameters are different.
Writing tests
After we wrote the tests, we can go ahead and build the actual calculator methods and once we've finished, all the tests should pass. Also, you can write a single method and run the tests to see what you're missing and then continue with the other.
tests/calculator.js
*Calculator methods*/(function(window) {// Calculator object var calculator = { // Sums two numbers sum: function(a, b) {return a + b;} // Subtract `b` to `a`, subtract: function(a, b) {return a - b;}// Divide `a` by `b`,divide: function(a, b) {return a / b;} // Multiply `a` by `b`, multiply: function(a, b) {return a * b;} }; // Globalize calculator so that it can be // used in other methods. window.Calculator = calculator;})(this);
Running Tests
QUnit offers a graphical interface to view tests results in the browser, in order to view them just open the main test file within the example repository test/index.html
, here tests will run automatically.
On the other hand,
QUnit tests can be run using [Grunt]() which is a [NodeJS]() build tool. In order to run tests this way, you should install [NodeJs]() then cd into the example repository try-grunt
and run npm install
and grunt
. After doing so, you should see the tests results
Conclusion
Event though unit testing adds more work to be done in a project, it provides a solid base for the project to keep growing healthy as we can easily spot things that are causing issues and make sure that we don't break things as we add more code. On the other hand, unit testing can help us when refactoring our code as we make sure that even though code changes, the expected functionality remains.
Resources
If you haven't done so, go ahead and download the
example repository on GitHub, it contains the tests and functionality for the calculator
. In order to run the tests in the browser, just open tests/index.html
or if you want to use Grunt, then, cd
into repository and run npm install
and grunt
.
To get you up and running, following you can find links for the websites of the technologies we went over.
Written by Pablo Vallejo
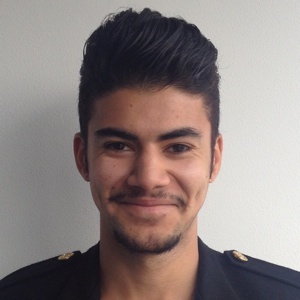
Pablo develops and optimizes software solutions, focusing on functionality and user experience. His expertise in coding and problem-solving ensures the creation of efficient and reliable applications.